Hi guys, at a major point in the project, I'm now at the testing phase.
We have finished the program to the beta stage, which means we are at the stage of putting it into the car and seeing what happens. So hopefully next week it will be going into the jeep

.
If anyone is interested in the code, here it is.
Please bear in mind I haven't tested this in the jeep yet, but if anyone wants to have a go at the project, feel free. Any feedback I can get is good

/*******************************************************************************
Written by Fish, Logic by Big-G
*
* Tiptronic gearbox controllor for Jeep AW4.
* Changes up and down gears, provides torque conveter lockup and
* a 7 segment led display readout of which gear you are in.
*
*******************************************************************************/
// function prototypes
void power_up();
void power_down();
void chng_to( int );
void abend();
void flash_disp();
int get_gear();
void handle_event( int );
void tcu_ctrl( int );
void tcl_ctrl( int );
// Define our pins - modify to ease hardware layout
const int p_in_sol1 = A0;
const int p_in_sol2 = A1;
const int p_in_onoff = 2;
const int p_out_tcu = 3;
const int p_in_up = 4;
const int p_in_down = 5;
const int p_in_tcl = 6;
const int p_out_sol1 = 7;
const int p_out_sol2 = 8;
const int p_out_sol_tcl = 9;
const int p_out_leddp = 10;
const int p_out_led1 = 11;
const int p_out_led2 = 12;
const int p_out_led3 = 13;
// Events
const int ev_power_change = 1;
const int ev_changeup = 2;
const int ev_changedown = 3;
const int ev_tcl_change = 4;
// Other constants
const int boot_delay = 1000;
const int pwr_up_delay = 1000;
const long debounce_delay = 10;
const int tcl_delay = 250;
const int tcl_long_press = 2000;
// State variables
int running = 0;
int tcl_running = 0;
int current_gear = 0;
int current_up_state = 0;
int current_down_state = 0;
int current_on_state = 0;
int current_tcl_state = 0;
int last_up_state = 0;
int last_down_state = 0;
int last_on_state = 0;
int last_tcl_state = 0;
int last_tcl_on_time = 0;
int auto_tcl_mode = 0;
// switch debounce tracking
long last_debounce_time = 0;
void setup() {
// define pins as inputs or outputs
pinMode( p_in_sol1, INPUT );
pinMode( p_in_sol2, INPUT );
pinMode( p_in_onoff, INPUT );
pinMode( p_out_tcu, OUTPUT );
pinMode( p_in_up, INPUT );
pinMode( p_in_down, INPUT );
pinMode( p_in_tcl, INPUT );
pinMode( p_out_sol1, OUTPUT );
pinMode( p_out_sol2, OUTPUT );
pinMode( p_out_sol_tcl, OUTPUT );
pinMode( p_out_leddp, OUTPUT );
pinMode( p_out_led1, OUTPUT );
pinMode( p_out_led2, OUTPUT );
pinMode( p_out_led3, OUTPUT );
Serial.begin(9600);
Serial.print("Starting...\n");
// power-up steps
tcu_ctrl( 1 ); // turn on power to the TCU
delay( boot_delay ); // hang on a god-damned second
current_gear = get_gear();
}
void loop() {
int up_state = digitalRead( p_in_up );
int down_state = digitalRead( p_in_down );
int on_state = digitalRead( p_in_onoff );
int tcl_state = digitalRead( p_in_tcl );
if (
up_state != last_up_state
|| down_state != last_down_state
|| on_state != last_on_state
|| tcl_state != last_tcl_state
) {
last_debounce_time = millis();
}
if ( (millis() - last_debounce_time) > debounce_delay ) {
if ( up_state != current_up_state ) {
current_up_state = up_state;
if ( current_up_state ) {
handle_event( ev_changeup );
}
}
if ( down_state != current_down_state ) {
current_down_state = down_state;
if ( current_down_state ) {
handle_event( ev_changedown );
}
}
if ( on_state != current_on_state ) {
current_on_state = on_state;
if ( current_on_state != running ) {
handle_event( ev_power_change );
}
}
if ( tcl_state != current_tcl_state ) {
current_tcl_state = tcl_state;
auto_tcl_mode = 0;
if ( current_tcl_state ) { // momentary switch
last_tcl_on_time = millis();
handle_event( ev_tcl_change );
} else {
if ( ( millis() - last_tcl_on_time ) > tcl_long_press ) { // long press
// auto_tcl_mode = 1;
}
}
}
}
last_up_state = up_state;
last_down_state = down_state;
last_on_state = on_state;
last_tcl_state = tcl_state;
if ( (get_gear() != current_gear ) && running ) {
abend();
}
}
void handle_event( int ev ) {
switch(ev) {
case ev_changeup :
if ( ! running ) break;
switch( current_gear ) {
case 4 :
//flash_disp();
break;
default :
chng_to( ++current_gear );
break;
}
break;
case ev_changedown :
if ( ! running ) break;
switch( current_gear ) {
case 1 :
//flash_disp();
break;
default :
chng_to( --current_gear );
break;
}
break;
case ev_power_change :
if ( running ) {
power_down();
} else {
power_up();
}
break;
case ev_tcl_change :
if ( running ) {
tcl_running = ! tcl_running;
tcl_ctrl( tcl_running );
}
break;
}
}
void power_up() {
current_gear = get_gear();
chng_to( current_gear ); // set solenoids and LEDs
tcu_ctrl( 0 ); // kill the TCU
delay( pwr_up_delay ); // hang on a god-damned second
if ( get_gear() == current_gear ) {
running = 1;
} else {
abend();
}
}
void power_down() {
// kill power to the gear solenoids
digitalWrite( p_out_sol1, 0 );
digitalWrite( p_out_sol2, 0 );
tcl_ctrl( 0 ); // turn off the tcl
tcu_ctrl( 1 ); // turn on power to the TCU
// blank the display
digitalWrite( p_out_led1, 0 );
digitalWrite( p_out_led2, 0 );
digitalWrite( p_out_led3, 0 );
running = 0;
}
void chng_to( int new_gear ) {
tcl_ctrl( 0 );
delay( tcl_delay );
switch( new_gear ) {
case 1 :
// gear solenoids
digitalWrite( p_out_sol1, 1 );
digitalWrite( p_out_sol2, 0 );
// LED display
digitalWrite( p_out_led1, 1 );
digitalWrite( p_out_led2, 0 );
digitalWrite( p_out_led3, 0 );
break;
case 2 :
// gear solenoids
digitalWrite( p_out_sol1, 1 );
digitalWrite( p_out_sol2, 1 );
// LED display
digitalWrite( p_out_led1, 0 );
digitalWrite( p_out_led2, 1 );
digitalWrite( p_out_led3, 0 );
break;
case 3 :
// gear solenoids
digitalWrite( p_out_sol1, 0 );
digitalWrite( p_out_sol2, 1 );
// LED display
digitalWrite( p_out_led1, 1 );
digitalWrite( p_out_led2, 1 );
digitalWrite( p_out_led3, 0 );
break;
case 4 :
// gear solenoids
digitalWrite( p_out_sol1, 0 );
digitalWrite( p_out_sol2, 0 );
// LED display
digitalWrite( p_out_led1, 0 );
digitalWrite( p_out_led2, 0 );
digitalWrite( p_out_led3, 1 );
break;
}
if ( auto_tcl_mode ) {
delay( tcl_delay );
tcl_ctrl( 1 );
}
}
void abend() {
// kill power to the gear solenoids
digitalWrite( p_out_sol1, 0 );
digitalWrite( p_out_sol2, 0 );
tcl_ctrl( 0 ); // turn off the tcl
tcu_ctrl( 1 ); // turn on power to the TCU
flash_disp(); // warn the driver
delay( 1000 );
// blank the display
digitalWrite( p_out_led1, 0 );
digitalWrite( p_out_led2, 0 );
digitalWrite( p_out_led3, 0 );
running = 0;
}
int get_gear() {
//return 3;
Serial.print( analogRead( A0 ), DEC );
Serial.print( " - " );
Serial.print( analogRead( A1 ), DEC );
Serial.print( "n" );
int val_sol1 = analogRead( A0 ) > 500 ? 1 : 0;
int val_sol2 = analogRead( A1 ) > 500 ? 2 : 0;
switch ( val_sol1 + val_sol2 ) {
case 0 : return 4;
case 1 : return 1;
case 2 : return 3;
case 3 : return 2;
}
}
void flash_disp() {
// show '0' and flash dp
//digitalWrite( p_out_led1, 0 );
//digitalWrite( p_out_led2, 0 );
//digitalWrite( p_out_led3, 0 );
//running = 0;
digitalWrite( p_out_leddp, 1 );
delay(250);
digitalWrite( p_out_leddp, 0 );
delay(250);
digitalWrite( p_out_leddp, 1 );
delay(250);
digitalWrite( p_out_leddp, 0 );
delay(250);
digitalWrite( p_out_leddp, 1 );
delay(250);
digitalWrite( p_out_leddp, 0 );
delay(250);
digitalWrite( p_out_leddp, 1 );
delay(250);
digitalWrite( p_out_leddp, 0 );
delay(250);
}
void tcu_ctrl(int on_or_off) {
digitalWrite( p_out_tcu, on_or_off ? 0 : 1 );
}
void tcl_ctrl(int on_or_off) {
digitalWrite( p_out_sol_tcl, on_or_off );
digitalWrite( p_out_leddp, on_or_off );
tcl_running = on_or_off;
}
-----------------------------------------------------------------------------------------------------------------------------
Well.
Lifes been really busy recently. Had alot of stuff to sort out.
Ive been doing bits here and there with the project, but i had to spend a month fixing the jeep so i can actually get to testing this.
Im still pretty stuck on the solenoid driver side, but ive found a local source of 'tronics knowlage that im tapping into

So just to let you all know i havent forgotten about this, and ill leave you with a couple of picutres of how far ive got as of tonight

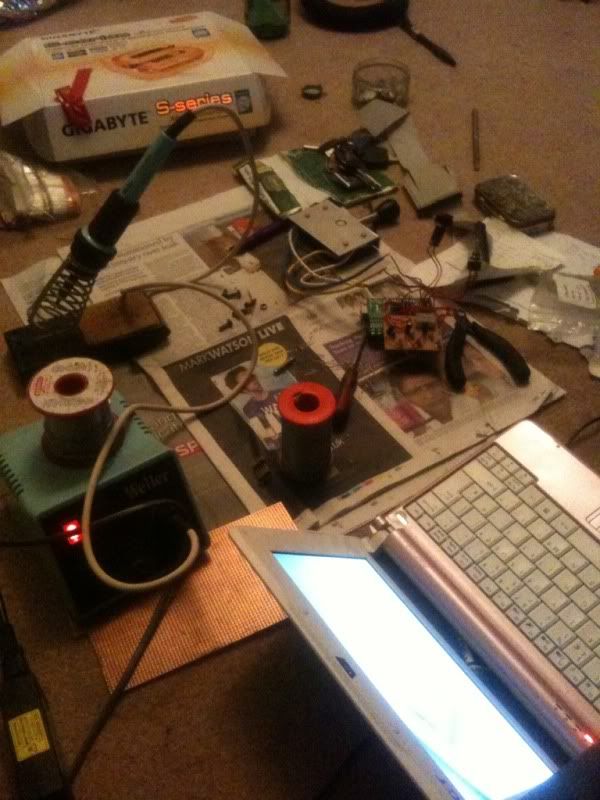
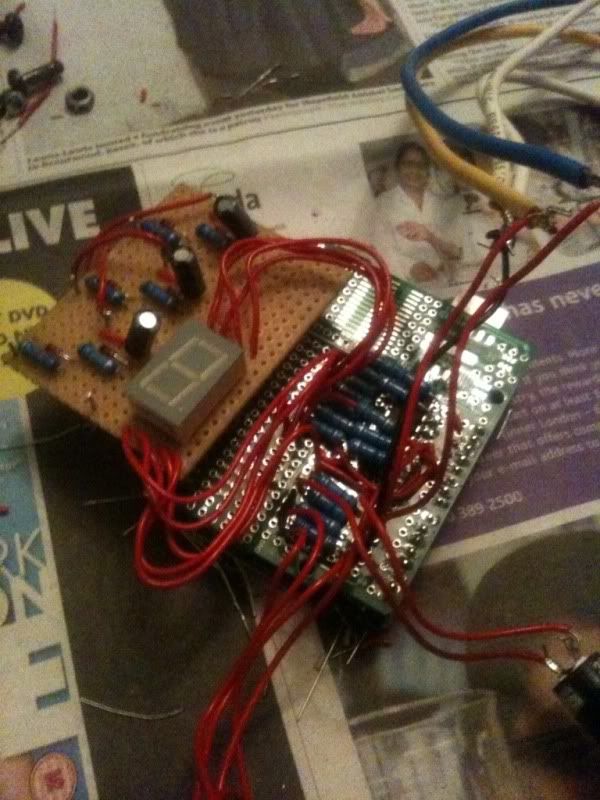
-----------------------------------------------------------------------------------------------------------------------------
Hello!
Sorry it's been so long. I got totally stuck on the high side driver, and after months of searching and trying things with mosfets and stuff I lost interest and banished it to a cupboard.
Recently I was pointed to a website called
http://ruggedcircuits.com/ which has a schematic for a high side driver.
I've ordered the parts and spent this evening bread boarding them and confirmed that it will work for what I want! Really pleased. Big thanks to rugged circuits.
I am currently designing the PCB in eagle, and this should be finished soon. Then it's off to veroboard for prototyping. I had to make some changes to the sensing side of the circuit, using optoisolators. This is yet to be tested, but I'm confident about it.
So all in all, I hope to have it prototyped in a couple of weeks, a bit of testing, and then a PCB made. Can't wait.
Watch this space
